MEAM.Design - MAEVARM - mMicroSD
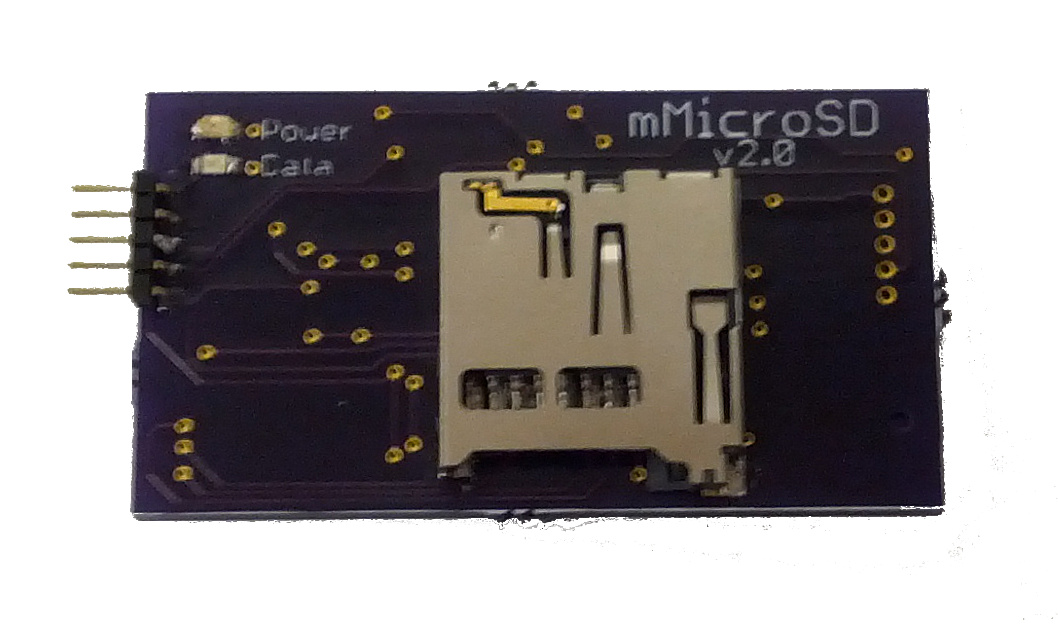
mMicroSD
The mMicroSD allows developers to read and write to a FAT32-formatted SD card over the mBus. Get the files here: mMicroSD_6.zip.
As of version 2.0, the mMicroSD peripheral can be directly plugged into the mBus header of both the M2 and M4.
Sample Program
A sample program for the M2, which provides a serial interface to view and edit files and directories, is available here: mMicroSD_sample_M2_v1.zip.
User Functions
Note: Long file names are NOT supported!
8.3 characters for files and 8 characters for directories MAX!
Startup and shutdown:
bool m_sd_init (void)
Mount the microSD card's FAT32 filesystem.bool m_sd_shutdown (void)
Commit any pending writes and unmount the filesystem.bool m_sd_commit (void)
Commit any pending writes (useful if you don't know when the system might be powered off)
File and directory information:
bool m_sd_get_size (const char *name, uint32_t *size)
Get the size of a file in the current directory.bool m_sd_object_exists (const char *name, bool *is_directory)
Search the current directory. If the target is found, *is_directory will be set accordingly.bool m_sd_get_dir_entry_first (char name[13], uint32_t *size, bool *is_directory)
bool m_sd_get_dir_entry_next (char name[13], uint32_t *size, bool *is_directory)
Iterate through the current directory, reading the names of its objects.
Returns false when the end of the directory has been reached.
If you don't need certain information, you can set those parameters to NULL.
Directory traversal and modification:
bool m_sd_push (const char *name)
Enter the directory with the given name.bool m_sd_pop (void)
Go up one level in the directory path.bool m_sd_mkdir (const char *name)
Create a subdirectory in the current directory.bool m_sd_rmdir (const char *name)
Remove an empty subdirectory from the current directory.bool m_sd_delete (const char *name)
Delete a file from the current directory. If you delete an open file, the file will be closed first.
File access and modification:
bool m_sd_open_file (const char *name, const open_option action, uint8_t *file_id)
Open a file in the current directory. Actions are:
READ_FILE
: Open an existing file as read-onlyAPPEND_FILE
: Open an existing file as read-write and seek to the endCREATE_FILE
: Create a file, deleting any previously existing file
You cannot open a file that is already opened elsewherebool m_sd_close_file (uint8_t file_id)
Close a file. Does nothing if the file is not open.bool m_sd_seek (uint8_t file_id, uint32_t offset)
Seek to a location within an opened file. An offset of FILE_END_POS
will seek to the end of the file.bool m_sd_get_seek_pos (uint8_t file_id, uint32_t *offset)
Get the seek position in the current file.bool m_sd_read_file (uint8_t file_id, uint32_t length, uint8_t *buffer)
Read from the current location in the file. Updates the seek position.
If the length of the read would go beyond the end of the file, an error is returned and nothing is read.bool m_sd_write_file (uint8_t file_id, uint32_t length, uint8_t *buffer)
Write to the current location in the file. Updates the seek position.
Error Codes
m_sd_error_code
Every mMicroSD function call returns a bool
to indicate whether it was successful (true
for success, false
for failure).
If a function fails, the reason why it failed will be stored in m_sd_error_code
, and can be one of these values:
ERROR_NONE
: No error
Card-level errors:
ERROR_RESET
: Error resetting the cardERROR_ENABLE_CRC
: Error enabling CRC checksumsERROR_INIT
: Error initializing the cardERROR_BLOCK_LENGTH
: Error setting the block lengthERROR_CARD_UNINIT
: The card has not been initialized yetERROR_NULL_BUFFER
: The read/write function was given a null bufferERROR_TOO_FAR
: Tried to read or write beyond the block length (512 bytes)ERROR_TIMEOUT
: Timeout when reading/writing to the cardERROR_CRC
: Too many CRC errors when reading/writingERROR_CACHE_FAILURE
: An error occurred in the block caching systemERROR_UNKNOWN
: Some other error
Filesystem-level errors:
ERROR_MBR
: Error reading MBRERROR_NO_FAT32
: No FAT32 filesystem found in the MBRERROR_FAT32_VOLUME_ID
: Unexpected values in the FAT32 volume IDERROR_FAT32_INIT
: FAT32 filesystem could not be mountedERROR_FAT32_CLUSTER_LOOKUP
: Bad value encountered when looking up a FAT32 entryERROR_FAT32_AT_ROOT
: Tried to pop directory when in the root directoryERROR_FAT32_NOT_FOUND
: The file or directory was not foundERROR_FAT32_NOT_DIR
: Tried to call push on a fileERROR_FAT32_END_OF_DIR
: Tried to read a directory entry after reaching the end of the directoryERROR_FAT32_NOT_FILE
: Tried to open a directory as a fileERROR_FAT32_NOT_OPEN
: Tried a read/write/seek operation on a file that isn't openERROR_FAT32_TOO_FAR
: Tried to read/seek beyond the file's lengthERROR_FAT32_ALREADY_EXISTS
: Tried to create a file or directory that already existsERROR_FAT32_FILE_READ_ONLY
: Tried to write to a file that was opened read-onlyERROR_FAT32_FULL
: No free space left on the cardERROR_FAT32_INVALID_NAME
: Passed a function a bad file or directory name (too long, invalid characters...)ERROR_FAT32_NOT_EMPTY
: Tried to call rmdir on an non-empty directoryERROR_FAT32_ALREADY_OPEN
: Tried to open a file that is already openERROR_FAT32_TOO_MANY_FILES
: Maximum number of files already openERROR_FAT32_BAD_FILE_ID
: Tried to use a file id higher than the maximum allowed
mBus-level errors:
ERROR_I2C_COMMAND
: Error communicating with the mMicroSD peripheral over I2CERROR_I2C_RESPONSE_TIMEOUT
: Timed out waiting for a response from the mMicroSD peripheralERROR_I2C_MESSAGE_TOO_LONG
: Tried to read or write too much data at a time
The code and schematic for the mMicroSD peripheral itself is here: mMicroSD_peripheral_3.zip