MEAM.Design - M4 - mTouch
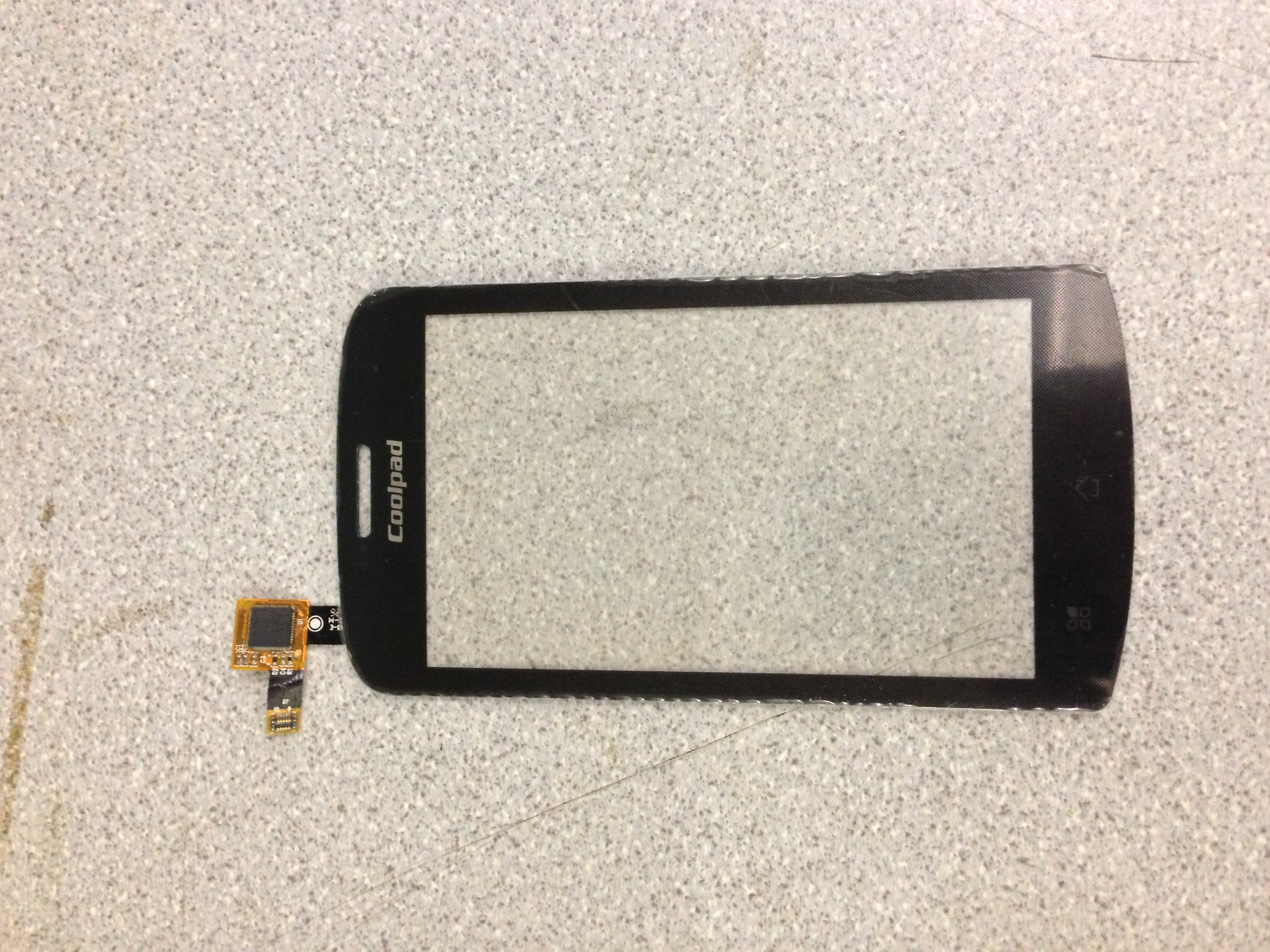
mTouch
The mTouch is for the use of Capacitive Touchscreen with M4. Developer is capable of setting up the Capacitive Touchscreen Module and attaining the position of the touch points. Users are able to do the multi-touch with mTouch which supports 5-point multi-touch. Download the files here: M4_mTouch.zip
Connections
4 pins are needed to make the connection between the mTouch peripheral and M4. The 4 pins are defined in the touch.h file and it allows users to make some changes to them. The default pins are PA3 which is connected with PEN, PA4 which is connected with TOUCH_SID, PA6 which is connected with TOUCH_SIC, PA5 which is connected with TOUCH_RST. PEN, TOUCH_SID, TOUCH_SIC and TOUCH_RST are on the peripheral.
- PA3 (Touch): PEN
- PA4 (I2C SID): TOUCH_SID
- PA6 (I2C SIC): TOUCH_SIC
- PA5 (Reset): TOUCH_RST
For the other 2 pins, one is VCC which should be connected with 3.3V, and the other one is GND which should be connected with ground.
Variables Definition
Note: Users cannot change the name of the following variables and, only when users add the touch.h in the project directory, users is in a position to use these useful variables directly.
_ts_event:
_ts_event is a structured type variable which contains 12 components(10 unsigned 16-bit variables to exhibit the positions of the touch points and 2 unsigned 8-bit variables).
- x1: the x-coordinate of the 1st Point
- y1: the y-coordinate of the 1st Point
- x2: the x-coordinate of the 2nd Point
- y2: the y-coordinate of the 2nd Point
- x3: the x-coordinate of the 3rd Point
- y3: the x-coordinate of the 3rd Point
- x4: the x-coordinate of the 4th Point
- y4: the x-coordinate of the 4th Point
- x5: the x-coordinate of the 5th Point
- y5: the x-coordinate of the 5th Point
- touch_point: the Number of Touch Points
- Key_Sta: the State of the Touch(0 means no touch and 1 means there are some touches.)
Note: Users have to use these variables in the following formation: ts_event.variable.
PEN( ):
PEN( ) is macro definition in touch.h which can be used to tell user if there are any touch on the screen.
- PEN( ) == 1: There are some touch.
- PEN( ) == 0: There is no touch.
Note: The variables are case-sensitive.
User Functions
void TOUCH_init (void)
Initialize the mTouch Module.void Pen_Int_Set (u8 en)
Open or close the interruption for touch.
- Pen_Int_Set(0); //Close the Interruption
- Pen_Int_Set(1); //Open the Interruption
u8 ft5x0x_read_data(void)
Get the coordinates of the touch points(maximum number of touch points is 5).
Functions for Advanced Application
I2C Communication between mTouch and M4:
void TOUCH_SID_OUT(void)
Set the I2C SID to be an output pin.void TOUCH_SID_IN(void)
Set the I2C SID to be an input pin.void TOUCH_Start(void)
Send an I2C start command to mTouch Module.void TOUCH_Stop(void)
Send an I2C stop command to mTouch Module.u8 TOUCH_Wait_Ack(void)
Wait for the I2C Acknowledgment Signal from mTouch Module. Return 1 if receive the Acknowledgement Signal successfully, or return 0.void TOUCH_Ack(void)
Generate an Acknowledgement Signal.void TOUCH_NAck(void)
Generate an non-Acknowledgement Signal.void TOUCH_Send_Byte(u8 txd)
Send 1 byte data to mTouch Module.u8 TOUCH_Read_Byte(unsigned char ack)
Read 1 byte data from mTouch Module. If ack == 0, generate an non-Acknowledgement Signal, or generate an Acknowledgement Signal. Finally, return what it has received.void TOUCH_RdParFrPCTPFun(u8 *PCTP_Par,u8 ValFlag)
Read data from mTouch Module starting from the 1st register in the module. *PCTP_Par is the location where you want to put the received data and ValFlag is the number of the data.
Example Code
Here is an example to use mTouch Module.
#include "mGeneral.h"
#include "mUSB.h"
#include "touch.h"
int main(void)
{
mInit( ); // Initialize the M4
TOUCH_Init( ); // Initialize the mTouch
mWait(1600000); // Delay for 100 ms
while(1)
{
if(ts_event.Key_Sta == Key_Down) // There is some touch on the screen
{
Pen_Int_Set(0); // Disable the interrupt
do
{
ft5x0x_read_data( ); // Read the coordinates of the touch points
ts_event.Key_Sta = Key_Up; // Update the status of the Key
}while(PEN( ) == 0); // If there is still some touch, run the loop again
Pen_Int_Set(1); // Enable the interrupt
}
}
}
Code for the mTouch peripheral: M4_mTouch.zip