MEAM.Design - MEAM 420 - P4: Slalom
Schedule
start: Tuesday, November 10th
team formation deadline: Monday, November 9th @ 6:00 p.m.
circle demonstration deadline: Tuesday, November 24th
slalom demonstration: Friday, December 11th
code submission: Friday, December 11th @ 11:59 p.m.
1. Team Formation
Before you will be able to get a robot, you will need to form a team with two other people. One person from your group must send an email to medesign@seas.upenn.edu with the title “420-P4-team” containing the names of the 3 people who will be working together. This email must be sent before 6:00 p.m. on Monday, November 9th. Anyone not included in a team email will be automatically assigned. A robot will be given to your team during class on Tuesday, November 10th.
2. Circle
Your robot will be placed 50 centimeters away facing a 10-cm tall, 2-cm diameter red pole. Once you start your script, your robot must trace out a 30-centimeter diameter circle around the red pole.
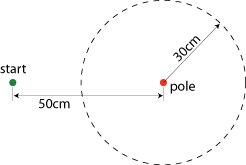
3. Slalom
How fast can your robot autonomously navigate it’s way through this three-gate slalom course?
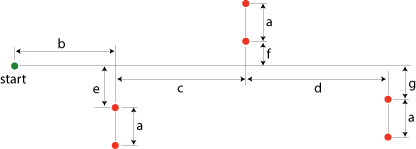
The intra-gate spacing, a, will be held constant at 20 cm, and the start distance, b, will be at least 30 cm. During the final competition the dimensions will be set the same for all teams. Once the timer starts, you cannot interact with your robot or make any changes on the computer to modify the performance of the robot. Your robot's score will be comprised of your total time plus penalties for missed and touched gates, where a missed gate will be a 60-second penalty and a touched gate will be a 15-second penalty.
4. Code Submission
Before the end of the day on Friday, December 11th, please send a copy of your team's Matlab code attached to an email to medesign@seas.upenn.edu entitled 420-P4-Code.
Getting Images from the onboard camera
Basics: http://fling.seas.upenn.edu/~cse390/wiki/?n=Main.Hardware
Fast Frame Server: http://fling.seas.upenn.edu/~cse390/wiki/?n=Software.FastFrameServer
Mobile Robot Control via Matlab
To command wheel velocities from Matlab, you'll need to download these files:
Extract the files and move them into your working directory for this project. You will now have access to four functions that should be used in the following manner:
1. Connect a dongle to your computer and wait for the system to find it. If you're using Windows, you may need to download these drivers (it should work out of the box on Mac or Linux) first.
2. Find the serial port identifier for the dongle. In Windows, open the device manager in the control panel, expand Ports, and find the COM# associated with the MaEvArM. On a Mac, run "ls /dev/tty.*" in terminal and note the usbmodem number.
3. Call the following function to open the serial port and configure the link to the robot
ROBOT = open_robot(PORT, NAME)
PORT
is the string identifier for your serial port (either 'COM#', or '/dev/tty.usbmodem#')
NAME
is the name of the robot you are using
ROBOT
is the handle for the serial port - you can name it anything you'd like
4. To set the wheel speeds, use:
set_velocities(ROBOT,L,R)
ROBOT
is the handle returned from open_robot
L
is the left-wheel speed between -999 (full reverse) and 999 (full forward)
R
is the right-wheel speed between -999 (full reverse) and 999 (full forward)
5. (Optional) To modify the velocity-loop gains, use:
set_gains(ROBOT,P,I,D,F)
ROBOT
is the handle returned from open_robot
P, I, D, F
are the proportional, integral, derivative, and feedforward controller gains, entered as values between -99.9 and 99.9
6. When you are finished you need to close the serial port:
close_robot(ROBOT)
ROBOT
is the handle returned from open_robot
For example:
myrobot = open_robot('/dev/tty.usbmodem411','beard');
set_velocities(myrobot,999,-999); % spin in place
pause(2); % wait for 2 seconds
set_velocities(myrobot,0,0); % full stop
close_robot(myrobot);
Modifying the Robot Code
If you're feeling brave, you're welcome to modify the embedded code on the robot itself. The files you will need are here: